Creating Meshes
Qkmaxware.Geometry takes a simple approach to 3D mesh data. Mesh geometry is represented as a collection of triangles in which each triangle is defined by 3 vertices in 3D space. The Qkmaxware.Geometry.ListMesh
class is the base class for most of the operations for 3D geometry.
Triangles Upon Triangles
Since mesh based geometry is just a collection of triangles, creating a mesh can be done by using the ListMesh
constructor with any IEnumerable<Triangle>
collection. Once created, a mesh is immutable. Alternatively, create any class that inherits from the IMesh
interface.
using System.Collections.Generic;
using Qkmaxware.Geometry;
public class Program {
public static void Main(string[] args) {
var tris = new List<Triangle>();
// ... add triangles
var mesh = new ListMesh(tris);
}
}
Primitive Generators
Since creating geometry using lists of triangles can be tedious, several generators are provided to quickly create primitive geometry within the Qkmaxware.Geometry.Primitives
namespace. Simply construct an object of the given type to automatically generate the required triangles.
Plane
Planes are 2d flat surfaces composed of two triangles, upper and lower. The geometry created by the Plane
generator is positioned in the XY plane with a normal pointed in the positive up (Z) direction.
Constructors
/// <param name="size">plane size</param>
/// <param name="centre">centre</param>
public Plane (double size, Vec3 centre)
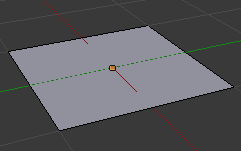
Cube
Cubes are 3d objects composed of 6 sides each side having 2 triangles for a total of 12 triangles.
Constructors
/// <param name="size">size of the cube</param>
/// <param name="centre">centre of the cube</param>
public Cube (double size, Vec3 centre)
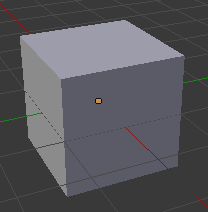
Cylinder
A cylinder is a 3D solid with circular end caps perpendicular to the axis. The Cylinder
generator allows for specifying different sized end caps.
Constructors
/// <summary>
/// Cylinder with different radii for top and bottom caps
/// </summary>
/// <param name="upperRadius">top cap radius</param>
/// <param name="lowerRadius">bottom cap radius</param>
/// <param name="height">height</param>
/// <param name="centre">centre</param>
/// <param name="resolution">subdivision level</param>
public Cylinder (double upperRadius, double lowerRadius, double height, Vec3 centre, int resolution = 8)
/// <summary>
/// Cylinder with uniform radius for top and bottom caps
/// </summary>
/// <param name="radius">radius</param>
/// <param name="height">height</param>
/// <param name="centre">centre</param>
/// <param name="resolution">subdivision level</param>
public Cylinder (double radius, double height, Vec3 centre, int resolution = 8)
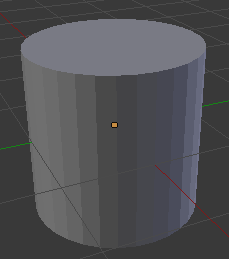
Tube
Tubes are cylindrical objects which are hollowed out along the vertical axis. The inner and outer radius allow for specifying the thickness of the tube's walls.
Constructors
/// <summary>
/// Cylinder with different radii for top and bottom caps
/// </summary>
/// <param name="outerRadius">outer radius</param>
/// <param name="innerRadius">inner radius</param>
/// <param name="height">height</param>
/// <param name="centre">centre</param>
/// <param name="resolution">subdivision level</param>
public Tube (double outerRadius, double innerRadius, double height, Vec3 centre, int resolution = 8)
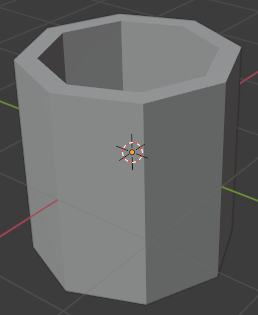
Caps
A capsule or "stadium of revolution" is a 3D geometric shape which is composed of a cylindrical body with two hemispheres for the top and bottom caps.
Constructors
/// <param name="radius">capsule hemisphere radius</param>
/// <param name="height">capsule total height</param>
/// <param name="centre">centre of the capsule</param>
/// <param name="horizontalResolution">longitude subdivision levels</param>
/// <param name="verticalResolution">latitude subdivision level</param>
public Capsule(double radius, double height, Vec3 centre, int horizontalResolution = 8, int verticalResolution = 8)
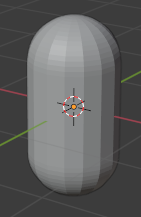
Cone
A cone is a 3D shape that tapers from a smooth flat shape to an apex point.
Constructors
/// <param name="radius">radius</param>
/// <param name="height">height</param>
/// <param name="centre">centre of the cone</param>
/// <param name="resolution">subdivision level</param>
public Cone (double radius, double height, Vec3 centre, int resolution = 8)
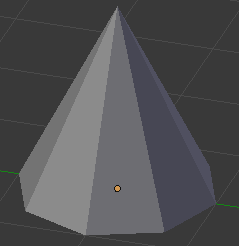
Sphere
The Sphere
generator can create spheres of different radius, given that geometry is represented by flat triangles, a sphere cannot be accurately represented. Instead, spheres are approximated using subdivision resolutions.
Constructors
/// <param name="radius">radius</param>
/// <param name="centre">centre point</param>
/// <param name="horizontalResolution">longitude subdivision levels</param>
/// <param name="verticalResolution">latitude subdivision level</param>
public Sphere(double radius, Vec3 centre, int horizontalResolution = 8, int verticalResolution = 8)
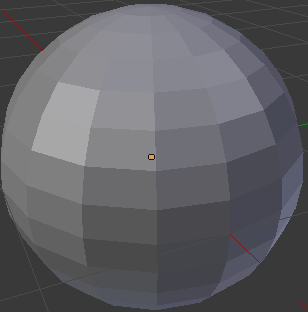
Hemisphere
Hemispheres are generated as the top half of a sphere plus a flat bottom face.
Constructors
/// <param name="radius">radius</param>
/// <param name="centre">centre point</param>
/// <param name="horizontalResolution">longitude subdivision levels</param>
/// <param name="verticalResolution">latitude subdivision level</param>
public Hemisphere(double radius, Vec3 centre, int horizontalResolution = 8, int verticalResolution = 8)
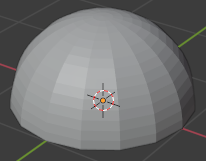
Torus
A torus is a surface of revolution generated by revolving a circle in 3D space. This shape is similar to inner tubes. Similar to the issues with spheres, a torus cannot be completely represented with triangular geometry and instead is approximated using the resolution and segments parametres.
Constructors
/// <param name="majorRadius">radius of the torus</param>
/// <param name="minorRadius">radius of the ring</param>
/// <param name="centre">centre of the torus</param>
/// <param name="resolution">quality of the rings</param>
/// <param name="segments">number of subdivisions on the ring</param>
public Torus (double majorRadius, double minorRadius, Vec3 centre, int resolution = 8, int segments = 8)
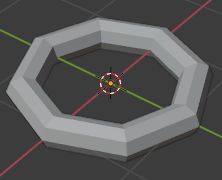
Frustum
The Frustum
generator creates right-frustum shapes which are parallel truncation cuts of a polygonal pyramid shape.
Constructors
/// <param name="size">size of the cube</param>
/// <param name="centre">centre of the cube</param>
public Frustum (double radius, double height, double ratio, Vec3 centre, int resolution = 4)
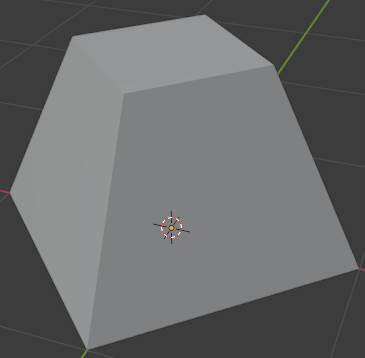
Nosecone
Aerodynamic nosecones represent a wide variety of shapes and the Nosecone generator can create several of the common nosecone shapes.
Constructors
/// <param name="radius">radius of the cone</param>
/// <param name="height">height of the cone</param>
/// <param name="resolution">higher number is more circular</param>
/// <returns>conic nosecone</returns>
public ConicNosecone (double radius, double height, int resolution = 8);
/// <param name="coneRadius">radius of the upper cone</param>
/// <param name="coneLength">length of the upper cone</param>
/// <param name="frustumRadius">radius of the lower conic frustum</param>
/// <param name="frustumLength">length of the lower conic frustum</param>
/// <param name="resolution">higher number is more circular</param>
/// <returns>biconic nosecone</returns>
public BiConicNosecone(double coneRadius, double coneLength, double frustumRadius, double frustumLength, int resolution = 8);
/// <param name="radius">nosecone radius</param>
/// <param name="height">nosecone height</param>
/// <param name="resolution">higher number is more circular</param>
/// <param name="segments">higher number is more smooth</param>
/// <returns>Tangent Ogive Nosecone</returns>
public TangentOgiveNosecone(double radius, double height, int resolution = 8, int segments = 8);
/// <param name="ogiveRadius">radius of the circle used to define the secant ogive</param>
/// <param name="conicRadius">base radius</param>
/// <param name="height">nosecone height</param>
/// <param name="resolution">higher number is more circular</param>
/// <param name="segments">higher number is more smooth</param>
/// <returns>Secant Ogive Nosecone</returns>
public SecantOgiveNosecone(double ogiveRadius, double conicRadius, double height, int resolution = 8, int segments = 8);
/// <param name="radius">radius of the nosecone</param>
/// <param name="height">height of the nosecone</param>
/// <param name="resolution">higher number is more circular</param>
/// <param name="segments">higher number is more smooth</param>
/// <returns>Elliptical Nosecone</returns>
public EllipticalNosecone(double radius, double height, int resolution = 8, int segments = 8);
/// <param name="K">parabolic parametre between 0 and 1</param>
/// <param name="radius">radius of the nosecone</param>
/// <param name="height">height of the nosecone</param>
/// <param name="resolution">higher number is more circular</param>
/// <param name="segments">higher number is more smooth</param>
/// <returns>Parabolic Nosecone</returns>
public ParabolicNosecone(double K, double radius, double height, int resolution = 8, int segments = 8);
/// <param name="K">power series parametre between 0 and 1</param>
/// <param name="radius">radius of the nosecone</param>
/// <param name="height">height of the nosecone</param>
/// <param name="resolution">higher number is more circular</param>
/// <param name="segments">higher number is more smooth</param>
/// <returns>Power Series Nosecone</returns>
public PowerseriesNosecone (double n, double radius, double height, int resolution = 8, int segments = 8);
/// <param name="K">Haack series parametre between 0 and 1</param>
/// <param name="radius">radius of the nosecone</param>
/// <param name="height">height of the nosecone</param>
/// <param name="resolution">higher number is more circular</param>
/// <param name="segments">higher number is more smooth</param>
/// <returns>Haack Series Nosecone</returns>
public HaackNosecone (double C, double radius, double height, int resolution = 8, int segments = 8);
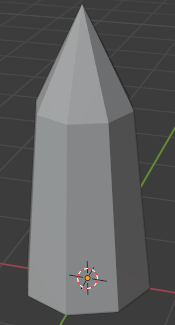
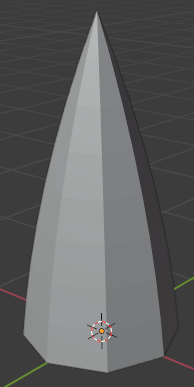
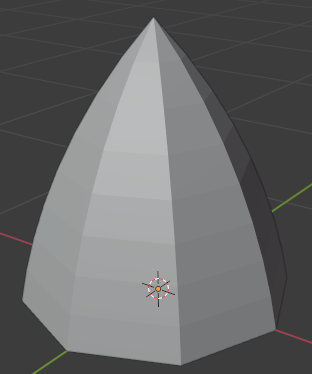
Arrow
Arrows are pointers looking in the +Z direction.
Constructors
/// <param name="length">length of the arrow</param>
/// <param name="radius">radius of the arrow head</param>
/// <param name="resolution">subdivision level</param>
public Arrow(double length = 1, double radius = 0.08, int resolution = 8)
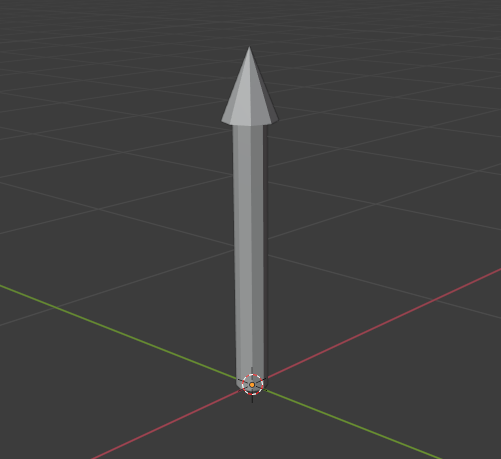
TextMesh
TextMesh creates meshes from string data by converting each letter to a 3G geometry. Custom fonts are used to map each character to their appropriate geometry.
Constructors
/// <param name="text">text</param>
public TextMesh (string text);
/// <param name="font">font</param>
/// <param name="text">text</param>
public TextMesh(Font3 font, string text)
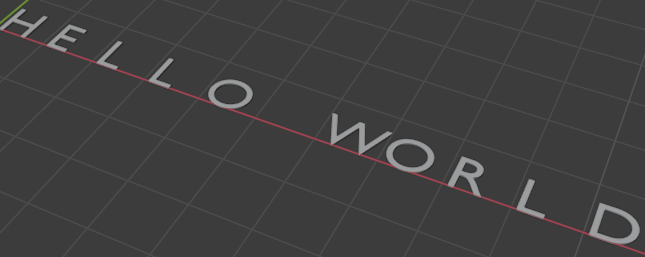